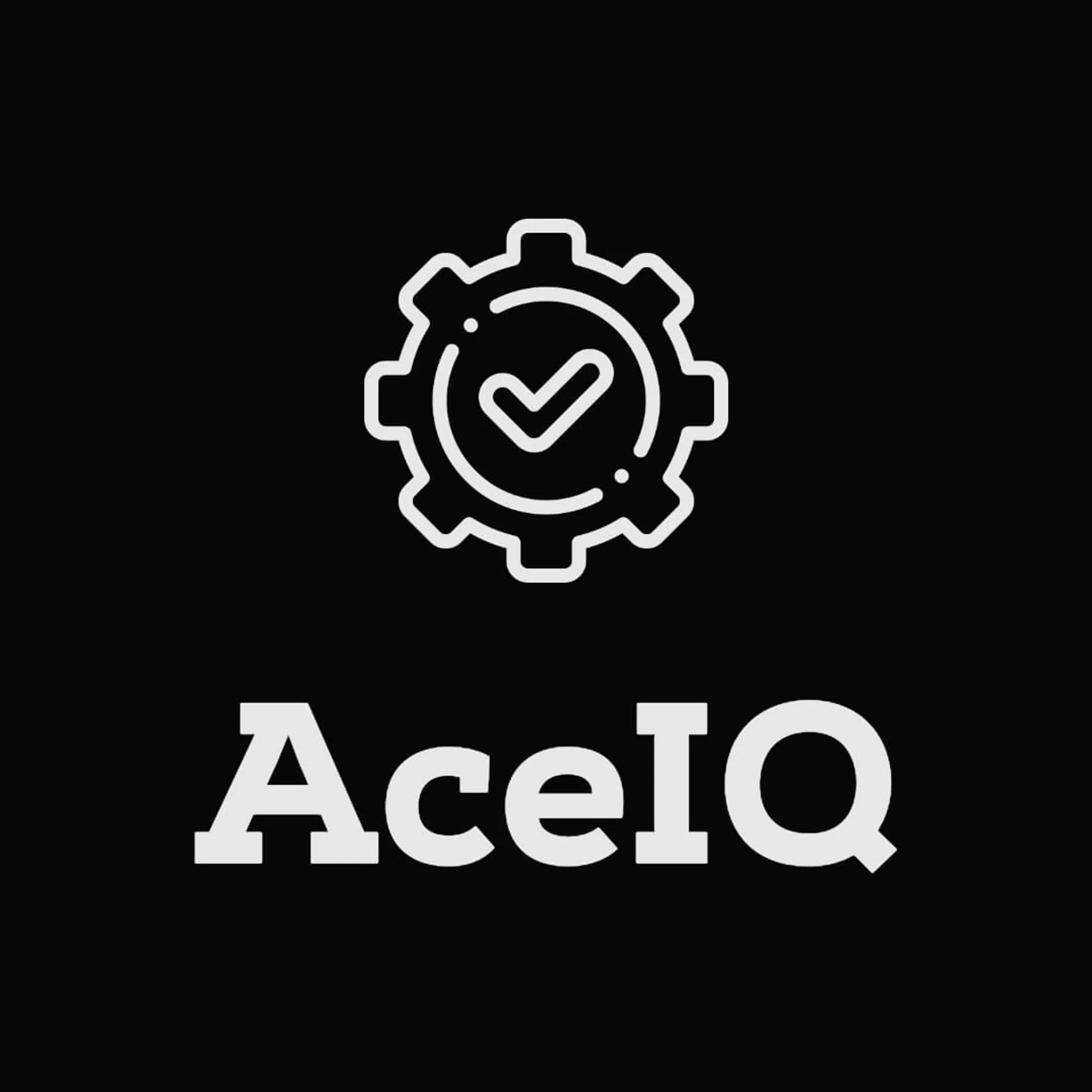
Are you looking to learn how to code a website with Python Flask? Look no further! In this tutorial, we will go over the basics of creating a website with Python Flask.
First, let’s go over the requirements for this tutorial:
To start, we will need to install Flask. You can do this by running the following command in your terminal:
pip install Flask
Once Flask is installed, we can start by creating a new Python file for our Flask app. Let’s call it app.py
.
Next, we will import Flask and create a new Flask app:
from flask import Flask
app = Flask(__name__)
Now that we have our Flask app, we can start adding routes. A route is a URL path that the user can visit in their web browser. For example, if we have a route at /hello
, the user can visit http://localhost:5000/hello
in their web browser to access it.
Let’s start by creating a simple “Hello, World!” route:
@app.route("/hello")
def hello():
return "Hello, World!"
In the code above, we used the @app.route
decorator to define a new route at /hello
. Then, we defined a function called hello()
that returns the string “Hello, World!”.
Now, we can run our Flask app by using the following code:
if __name__ == "__main__":
app.run()
To test our Flask app, we can run the following command in our terminal:
python app.py
This will start the Flask development server and you can visit http://localhost:5000/hello
in your web browser to see the “Hello, World!” message.
That’s it! You now know the basics of creating a website with Python Flask. Of course, there is much more you can do with Flask, such as adding more routes, rendering templates, and handling forms. But this should give you a good starting point.
Happy coding!